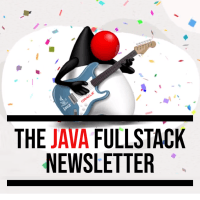
🎬 Java Record Classes
Modeling Simple Data with French Cinema Flair
In Java, record classes are a special kind of class introduced to model plain data aggregates with much less boilerplate than traditional classes (introduced in Java 16).
They’re ideal when you just want to group data together — like a name and age of an actor — without writing a bunch of repetitive code.
📚 For a deep dive, check out JEP 395.
🎭 What Is a Record?
A record automatically generates:
🟣 private final fields,
🟣 accessor methods (getters),
🟣 a constructor,
🟣 sensible equals(), hashCode(), and toString() implementations.
Because of this, a record is immutable and behaves like a concise data carrier.
🎬 Example: A French Actor
Let’s say we want to represent a famous French actor with their name and birth year. Here's the short and sweet version using a record:
This is equivalent to writing the following verbose class:
🧠 Under the Hood: What Gets Generated?
When you write:
Java generates:
🟣 Two private final fields: name and birthYear
🟣 Two accessor methods: name() and birthYear()
🟣 A constructor: new Actor(name, birthYear)
🟣 A smart equals() and hashCode() implementation
🟣 A toString() like: Actor[name=Jean Dujardin, birthYear=1972]
🎬 Using a Record in Action
🖨️ Output:
You can also compare records easily:
✨ Summary
Java record classes are perfect for creating immutable data containers — especially useful when dealing with DTOs, results from APIs, or any simple grouping of values. Plus, with records, your code stays clean and expressive — like a well-written script!