🛣️☕2️⃣1️⃣ Dear followers, let's prepare for Java 21 certification together!
1️⃣ How would you answer this question:
Given:
What is printed?
A) 0
B) 1
C) 2
D) An exception is thrown at runtime.
E) Compilation fails.
#PathToJava21 #java #certification
💡 Solution
Let's analyze the behavior of the code.
* Understanding HashSet
- HashSet in Java does not allow duplicate elements.
- It determines uniqueness using both hashCode() and equals().
* Examining Bottle Class
1. equals(Object o) Implementation:
public boolean equals( final Object o ) {
return Objects.equals( name, this.name );
}
- This implementation is incorrect because it does not properly compare the fields of Bottle.
- It only checks if the name of the incoming object is equal to this.name, but it does not compare the cl field.
- It also has a logical flaw: it does not check whether o is an instance of Bottle before casting.
2. hashCode() Implementation:
public int hashCode() {
return cl;
}
- The hashCode() method only uses cl to generate a hash code, ignoring the name field.
* Adding Bottles to HashSet
We execute:
bottles.add( new Bottle( "Champagne", 75 ) );
bottles.add( new Bottle( "Champagne", 300 ) );
- The first Bottle("Champagne", 75):
hashCode() returns 75.
No previous element with hash 75, so it gets added.
- The second Bottle("Champagne", 300):
hashCode() returns 300.
No previous element with hash 300, so it gets added.
Since the two Bottle instances have different hash codes (75 and 300), they are treated as different objects, even though the equals() method is faulty.
* Expected Output
Both Bottle instances are added successfully.
bottles.size() returns 2.
* Correct Answer:
✅ C) 2
(Working with Arrays and Collections)
2️⃣ How would you answer this question:
Given:
What is printed?
A) [200000, 500000, 900000, 2100000]
B) [100000, 400000, 1000000, 2200000]
C) [Lille, Lyon, Marseille, Paris]
D) An exception is thrown at runtime.
E) Compilation fails.
#PathToJava21 #java #certification
💡 Solution
Let's analyze the given code step by step:
* Step 1: Initialize the TreeMap
- A TreeMap is created and initialized with city names as keys and population numbers as values.
- Since TreeMap is sorted by keys (natural order for String), the map will store:
{ Lille=200000, Lyon=500000, Marseille=900000, Paris=2100000 }
* Step 2: Modify Values with replaceAll
- If the city name starts with "L", subtract 100,000 from the population.
- Otherwise, add 100,000.
Applying this logic:
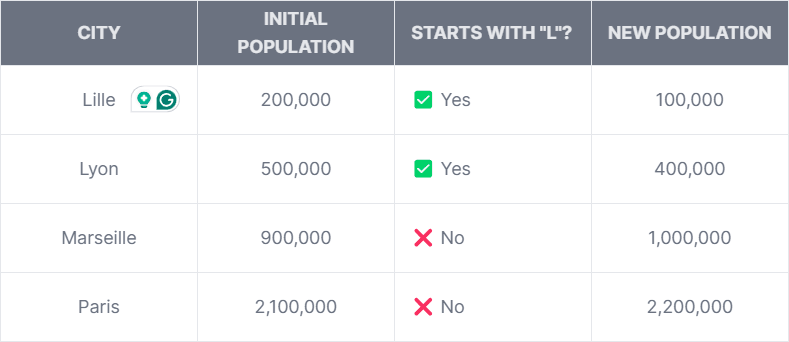
So, after replaceAll, the TreeMap contains:
{ Lille=100000, Lyon=400000, Marseille=1000000, Paris=2200000 }
* Step 3: Print Values
System.out.println(cityPopulation.values());
cityPopulation.values() returns a Collection of values from the TreeMap, preserving the sorted order of keys:
[100000, 400000, 1000000, 2200000]
* Step 4: Match with Options
The correct answer is:
B) [100000, 400000, 1000000, 2200000] ✅
(Working with Arrays and Collections)
3️⃣ How would you answer this question:
Given:
What is printed?
A) 5
B) 9
C) 1
D) An exception is thrown at runtime.
E) Compilation fails.
#PathToJava21 #java #certification
💡 Solution
Let's analyze the given Java code step by step.
Given Functions:
Function addTwo = i -> 2 + i;
Function tripleIt = i -> 3 * i;
- addTwo: Takes an integer i, adds 2, and returns the result.
Example: addTwo.apply(3) = 2 + 3 = 5
- tripleIt: Takes an integer i, multiplies it by 3, and returns the result.
Example: tripleIt.apply(3) = 3 * 3 = 9
* Composition:
Function calculateIt = addTwo.compose(tripleIt);
- compose means the function applied first is tripleIt, and the result is then passed to addTwo.
Thus, calculateIt.apply(x) is equivalent to:
addTwo.apply(tripleIt.apply(x))
* Calculation:
System.out.println(calculateIt.apply(1));
1. tripleIt.apply(1) → 3 * 1 = 3
2. addTwo.apply(3) → 2 + 3 = 5
* Answer:
The output is 5, so the correct answer is:
A) 5
(Working with Streams and Lambda expressions)
️4️⃣ How would you answer this question:
Given:
What is printed?
A) bonjour salut Coucou enchanté salut
B) bonjour salut Coucou enchanté
C) nothing
D) An exception is thrown at runtime.
E) Compilation fails.
#PathToJava21 #java #certification
💡 Solution
Let's analyze the given Java Stream code step by step:
* Understanding peek():
- The method peek(System.out::println) is used to print each element as it flows through the stream.
- However, streams in Java are lazy, meaning nothing happens until a terminal operation is applied (such as collect(), forEach(), count(), etc.).
- Since no terminal operation is present in the code, the stream is never consumed.
* Expected Output:
Since no terminal operation is called, no elements will be processed and nothing will be printed.
* Correct Answer:
✅ C) nothing
(Working with Streams and Lambda expressions)
5️⃣ How would you answer this question:
Given:
What is printed?
A) @FR#BE#CA$
B) #FR@BE@CA$
C) @#$FR@#$BE@#$CA@#$
D) An exception is thrown at runtime.
E) Compilation fails.
#PathToJava21 #java #certification
💡 Solution
Let's analyze the given Java code:
var frenchSpeakingCountries = Stream.of("FR", "BE", "CA");
var mix = frenchSpeakingCountries.collect(Collectors.joining("@", "#", "$"));
System.out.println(mix);
* Breakdown:
1. Stream.of("FR", "BE", "CA")
Creates a stream containing three elements: "FR", "BE", and "CA".
2. Collectors.joining("@", "#", "$")
The joining collector has three arguments:
- Delimiter: "@" (placed between elements)
- Prefix: "#" (placed at the start)
- Suffix: "$" (placed at the end)
This results in:
#FR@BE@CA$
3. Output:
System.out.println(mix);
Prints #FR@BE@CA$.
* Correct Answer:
✅ B) #FR@BE@CA$
(Working with Streams and Lambda expressions)
6️⃣ How would you answer this question:
Given:
and given the orders.csv file:
What is printed?
A) The lines of orders.csv.
B) The first line of orders.csv.
C) Nothing.
D) An exception is thrown at runtime.
E) Compilation fails.
#PathToJava21 #java #certification
💡 Solution
The given Java code attempts to read a file (orders.csv) and print its contents. Let's analyze each step:
* File Creation
var ordersFile = new File( "orders.csv" );
- This creates a File object representing the file orders.csv.
* Reading File Contents
var ordersLines = Files.readAllLines( ordersFile );
Files.readAllLines(Path) expects a Path object, but ordersFile is a File object.
- File does not have an implicit conversion to Path. The correct way would be:
var ordersLines = Files.readAllLines(ordersFile.toPath());
Because of this, compilation fails.
* Correct Answer:
E) Compilation fails.
(Using Java I/O API)