🔁 Understanding Kafka Serialization and Deserialization: A Guide to the Serde Object 🔁
🔁 Understanding Kafka Serialization and Deserialization: A Guide to the Serde Object 🔁
In the world of Apache Kafka, data streaming is the heartbeat of modern applications. But how does Kafka ensure that the data it sends and receives is understandable across different systems? The answer lies in serialization and deserialization, commonly known as Serde.
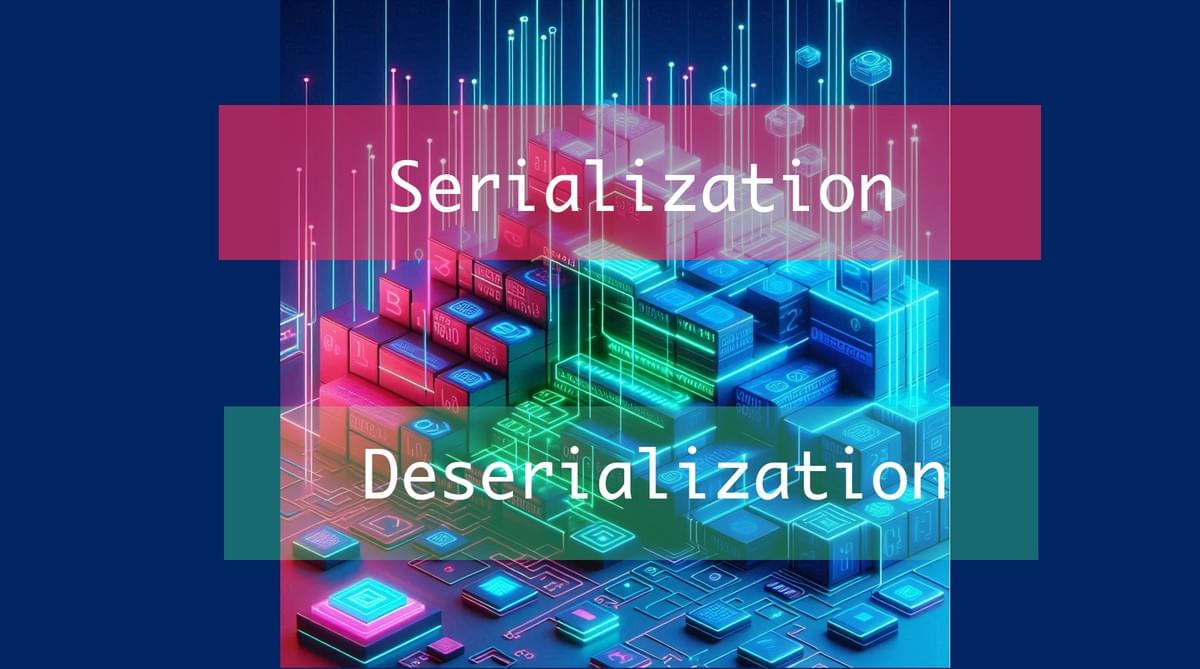
🔄 Serialization in Kafka Serialization is the process of turning structured objects into a byte stream for transmission over Kafka. It’s like packing your luggage into a suitcase before a flight; everything needs to fit in a compact, transportable form.
🔄 Deserialization in Kafka Deserialization is the reverse process, where byte streams are converted back into structured data. It’s like unpacking your suitcase after a journey, restoring items to their original state.
🛠️ The Serde Object In Kafka, a Serde object is a combination of a Serializer and a Deserializer. It’s a handy tool that allows developers to define how data should be transformed in both directions.
📊 When and Where is Serde Used? Serde comes into play whenever Kafka needs to understand the data it’s dealing with. This could be:
- When producing data to a Kafka topic.
- When consuming data from a Kafka topic.
- During Kafka Streams processing.
🔑 Key Takeaway Points
- Serde is essential for data integrity across systems.
- Custom Serde implementations allow for flexible data structures.
- Always match the Serde to the data type you’re working with.
- Use default Serdes for common data types like String, Integer, etc.
- For complex data types, consider implementing custom Serdes.
Remember, without proper serialization and deserialization, data streaming would be like trying to fit a square peg into a round hole. Serde makes sure everything fits just right! ✅
Here’s an example of a Kafka consumer using Serde for custom object deserialization:
and
In this code:
- We set up the properties for the Kafka consumer, including the bootstrap servers, group ID, and deserializers for both the key and value.
- We specify a custom deserializer for the value, which you would need to implement based on how your custom objects are serialized.
- We create a KafkaConsumer instance, subscribe to a topic, and start polling for records.
- The YourCustomDeserializer class is a placeholder for your custom deserialization logic.
Remember to replace "your.custom.Deserializer.class" with the actual class name of your deserializer and implement the deserialization logic in the deserialize method. This example assumes that the key is a String and the value is a custom object that you want to deserialize. If you have a different key type, you’ll need to use the appropriate Deserializer for that type as well.